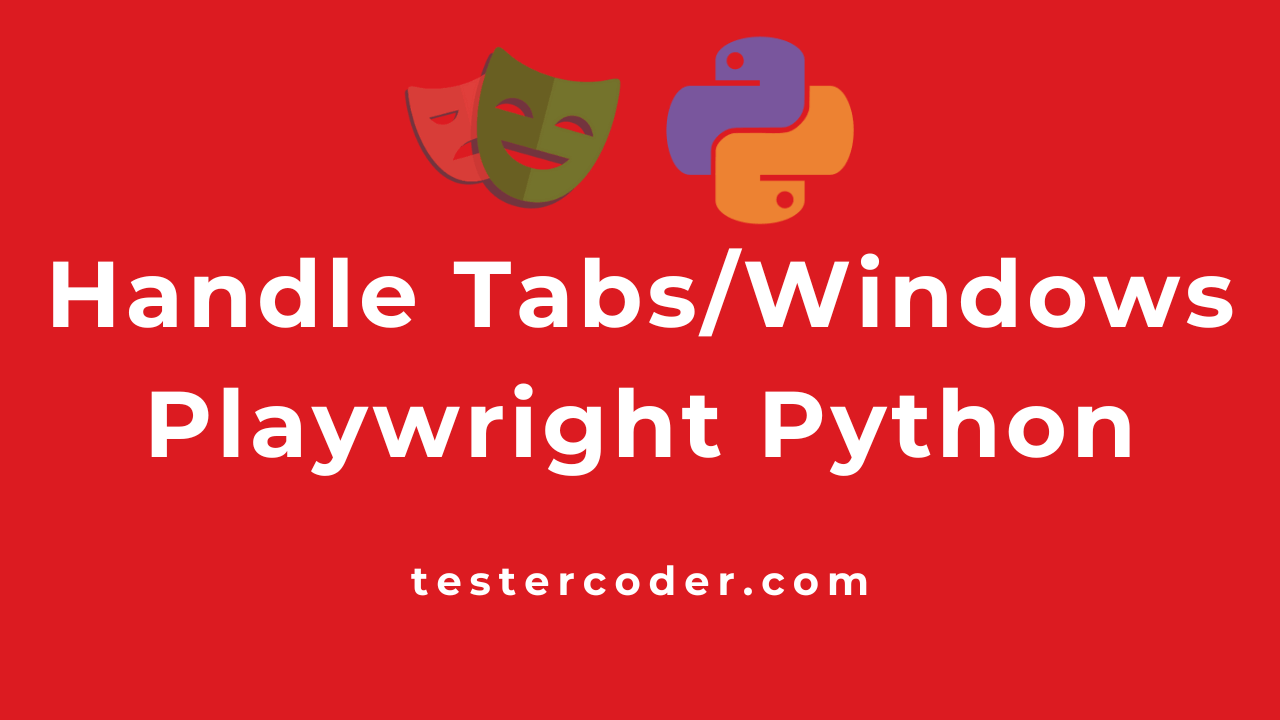
Handle Tabs and Windows in Playwright Python
In Playwright, we can handle the tabs/windows that opened during execution by waiting for a page to open, we will not be switching between tabs, instead, we will store the new tab in a variable and treat it just like page
.
We will use page.expect_page()
from Playwright to handle the tabs/windows, once we store the page in a new variable we can use both the new page and the old page simultaneously.
The below method works only if you know that the action will open a new tab.
from playwright.sync_api import sync_playwright, expect, Page
playwright = sync_playwright().start()
browser = playwright.chromium.launch(headless=False)
context = browser.new_context()
page = context.new_page()
page.goto("url")
with context.expect_page() as new_page_object:
page.locator("a .link").click() #action
new_page:Page = new_page_object.value
new_page.locator("#newelemnt").click()
page.locator("#oldelement").fill()
More than one page opens while clicking a link:
When click a link and more than one page opens, then page.expect_page() will not be efficient, in that case, use context.pages
- Create object page from Context than Browser object
- Open the website
- Click the link that opens multiple browsers/tabs (total should be more than 2, otherwise, use the above solution itself)
- Get all the pages/tabs on the context using
context.pages
- Iterate through each of the pages and compare the titles of each page, and break the loop when the page you looking is arrives; also store the page in a new variable so that you can use it further.
from playwright.sync_api import sync_playwright, expect, Page
playwright = sync_playwright().start()
browser = playwright.chromium.launch(headless=False)
context = browser.new_context()
page = context.new_page()
page.goto("url")
all_pages = context.pages
new_page = None
for new in all_pages:
if "target" in new.title():
new_page = new
break
new_page.locator("#element").click()
page.locator("#old_element").fill("some")
Not sure when tabs/windows opens
This is a bad design from the development team. Anyway, learn to handle it
If you are not sure when the new tab opens but want to handle it whenever it opens then you can use the page.on
method.
- Open the website
- Create a function and write code about what needs to be performed when a new page/window opens, This should be declared before using
page.on
- Trite page.on(“page”, handle_new_tab_or_window) and this is the function where you already declared what should happen
- Then write the code that clicks a link or performs some task that opens the new tab/browser window
from playwright.sync_api import sync_playwright, expect, Page
playwright = sync_playwright().start()
browser = playwright.chromium.launch(headless=False)
page = browser.new_page()
page.goto("url")
def handle_new_tab_or_window(page:Page):
page.locator("#new_element").click()
page.on("page", handle_new_tab_or_window)
page.locator("#link_that_opens_tabs").click()