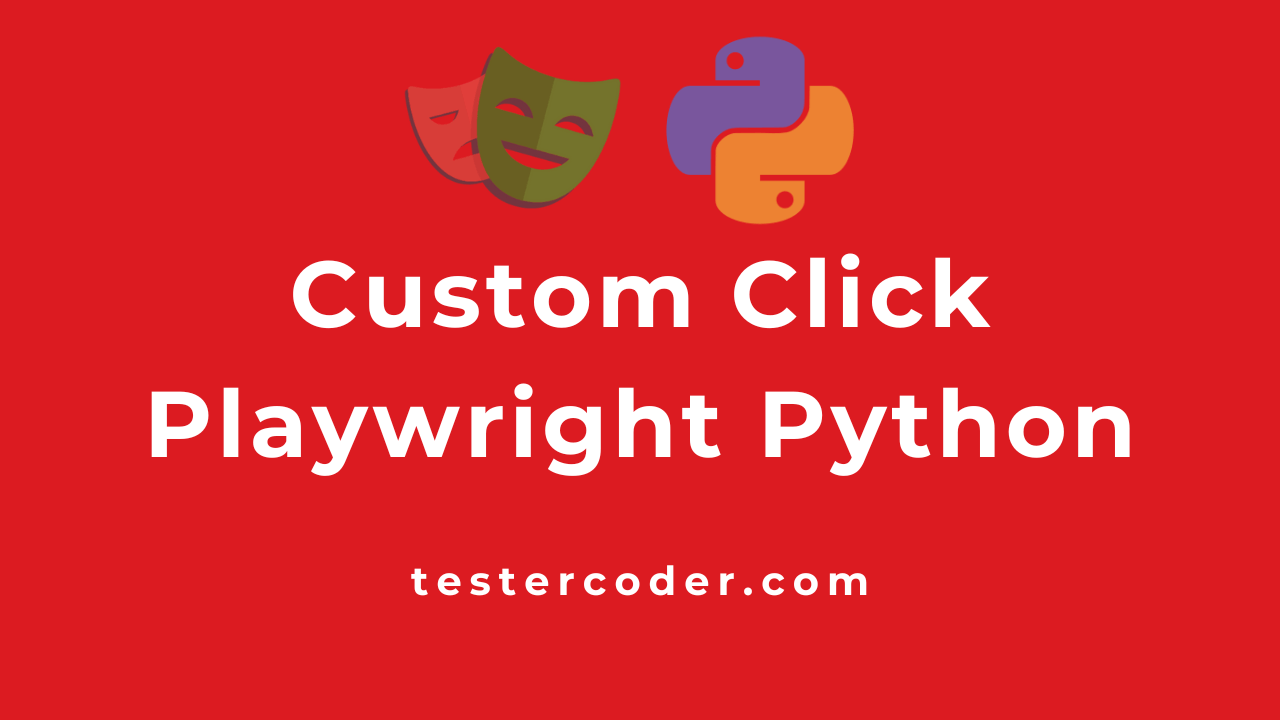
Click and custom click in Playwright Python
In the previous chapter, you have learned how to click an element in Playwright Python. If you have noticed a difference when you find an element and if the element has more than one match, then you will get an exception. But in other tools (like selenium), it normally clicks the first element it matches, but not in Playwright.
When you are creating a framework, you should be in a position to handle such kinds of imperfections. Even if there is more than one match present, you should always try to click the first match.
As the development proceeds, There is the chance locator can have multiple matches and every time you cannot change the index of the element so that the code can click the first element. So we will be learning a method/function to handle the click.
Below I am trying to click an element from the Google home page where I will have more than one match and I want to click the first match, here About is the first match
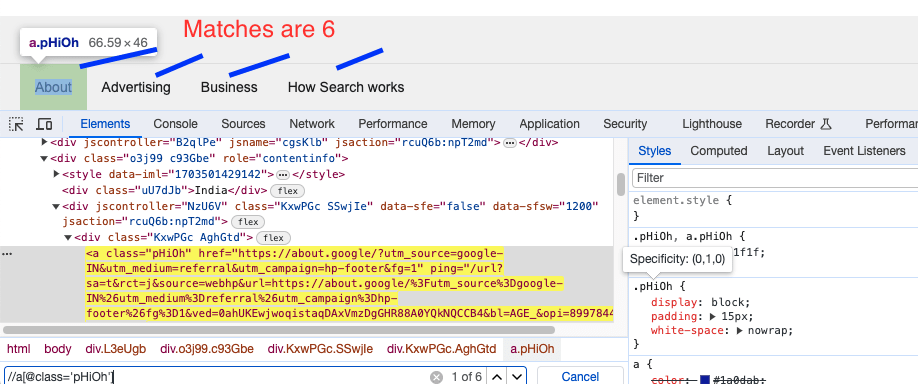
Normal Click
from time import sleep
from playwright.sync_api import sync_playwright
playwright = sync_playwright().start()
browser = playwright.chromium.launch(headless=False)
page = browser.new_page()
page.goto("https://google.com")
page.locator("//a[@class='pHiOh']").click()
The output is:
playwright._impl._api_types.Error: Error: strict mode violation: locator("xpath=//a[@class='pHiOh']") resolved to 6 elements:
1) <a class="pHiOh" href="https://about.google/?utm_so…>About</a> aka get_by_role("link", name="About")
2) <a class="pHiOh" href="https://www.google.com/intl/…>Advertising</a> aka get_by_role("link", name="Advertising")
Custom Click:
Instead of clicking the element, we will be writing a function where we will check how many matches are currently present. Based on that one, we will be clicking the element.
The function will accept the element as a parameter. Along with that I have added print statements, you can add logging statements as well.
from time import sleep
from playwright.sync_api import sync_playwright
playwright = sync_playwright().start()
browser = playwright.chromium.launch(headless=False)
page = browser.new_page()
page.goto("https://google.com")
def custom_click(element):
if element.count() == 1:
print("Only one element is present, clicking the element")
print("Clicking :", element)
element.click()
else:
print("More than one element is present, clicking the first element")
print("Clicking :", element.nth(0))
element.nth(0).click()
element_to_click = page.locator("//a[@class='pHiOh']")
custom_click(element_to_click)
Output:
/Users/pavan/PycharmProjects/ST/PlaywrightPython/bin/Python /Users/pavan/PycharmProjects/PlaywrightPython/custom-click.py
More than one element is present, clicking the first element
Clicking : <Locator frame=<Frame name= url='https://www.google.com/'> selector="//a[@class='pHiOh'] >> nth=0">
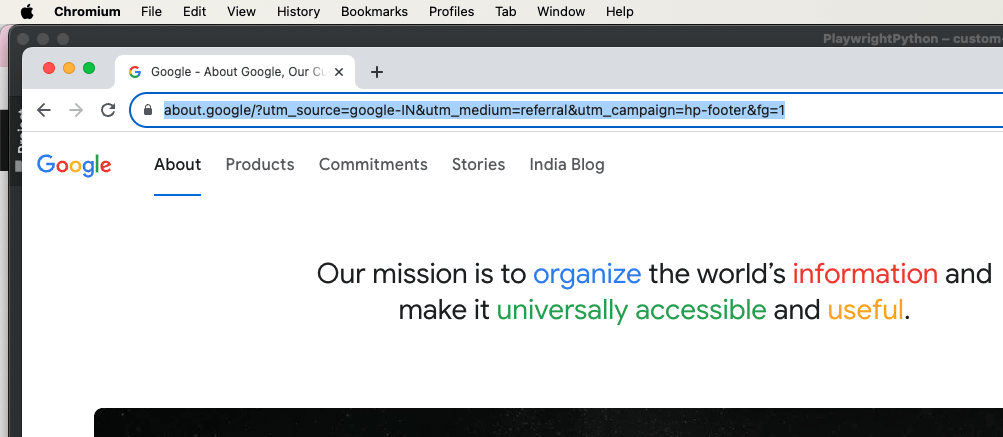