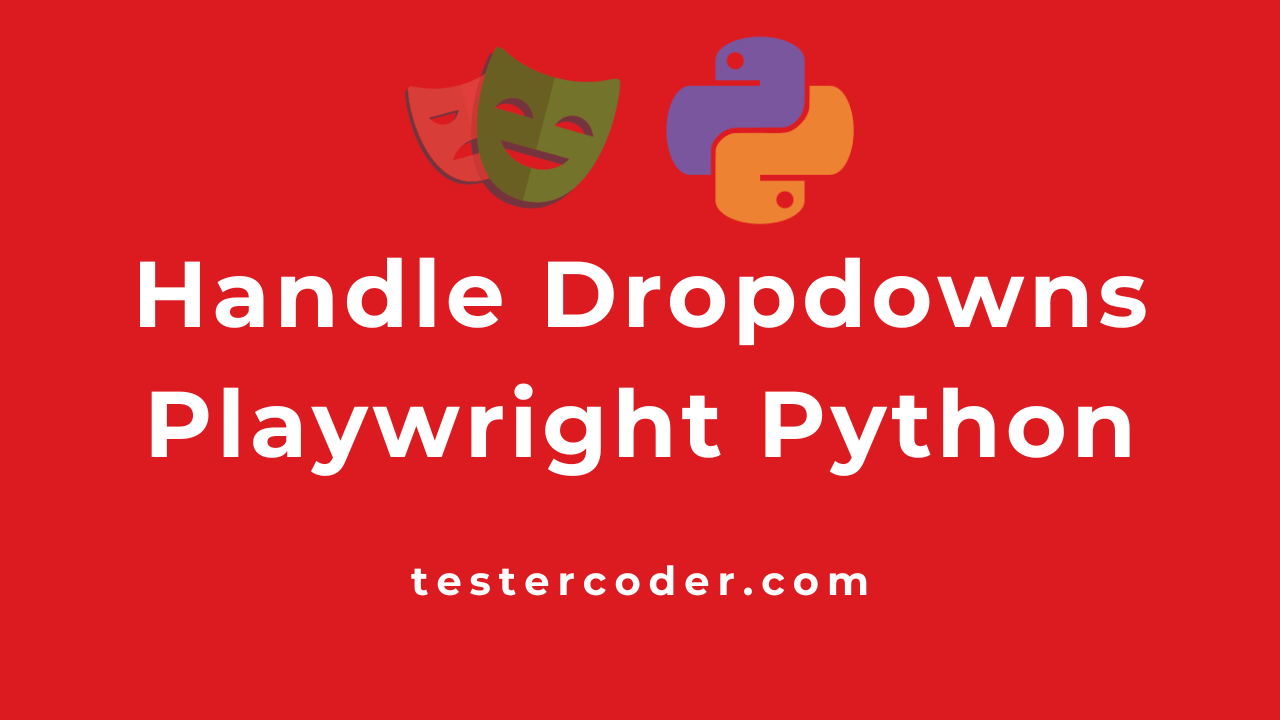
Handle dropdowns in Playwright Python
In this article, we will learn about automating dropdowns in Playwright. In general, dropdowns are categorized into two categories 1. Standard dropdown. 2. Custom dropdown.
- Standard Dropdown: formed using standard HTML tags (select tag), Playwright supports standard dropdowns out of the box
- Single-value dropdown
- Multi-value dropdown
- Custom Dropdown: formed using tools that might use different HTML tags, dropdown methods do not work with custom dropdowns
Standard dropdown:
Standard dropdown is formed using the select tag in HTML, You can handle the standard dropdown using methods/functions provided by Playwright. Again, you will have two categories standard dropdown.
Dropdown Details in Playwright:
- Index starts from 0 in dropdowns
- Value is a ‘value’ attribute (you can see something like this
value='abc'
) - Value could be different from Visible text(Note: In the below dropdown image, the value is Apple, but the Visible Text is ‘iPhone’ )
- Visible text is the text between ‘>’ and ‘<‘
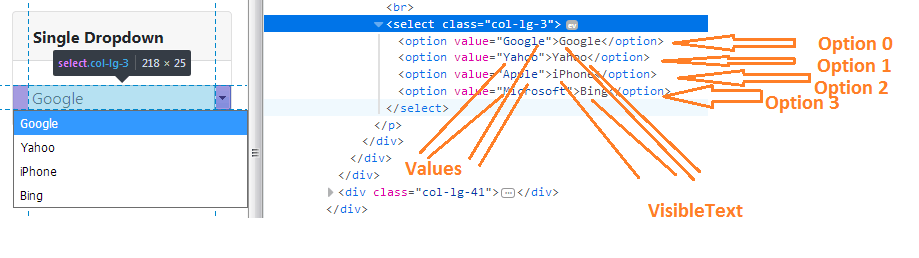
Single value dropdown:
You can select only one value from the dropdown.
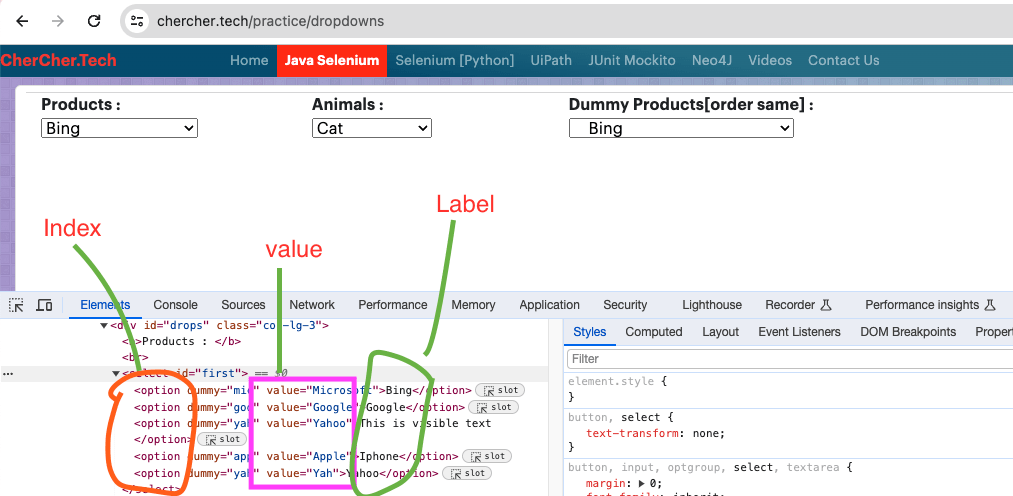
If you notice in the above image, There are three items highlighted, using these three items we can select a dropdown along with 4th (using element)
- Index: The options are numbered by default from 0, so Bing 0, Google 1, This is Visible text 2, and so on
- Value: mentioned with
value
attribute - Label: the text present/ visible to the user, Bing, Google, and so on
We will be using select_option
function to select a value from dropdowns. select_option
is case-sensitive, so whatever value/text is present on the dropdown you have to provide the same without changing the case. This method returns a list of options selected.
Select By Value with Playwright
from playwright.sync_api import sync_playwright
playwright = sync_playwright().start()
browser = playwright.chromium.launch(headless=False)
page = browser.new_page()
page.goto("https://chercher.tech/practice/dropdowns")
select_option = page.locator("//select[@id='first']").select_option(value="Yahoo")
print(select_option)

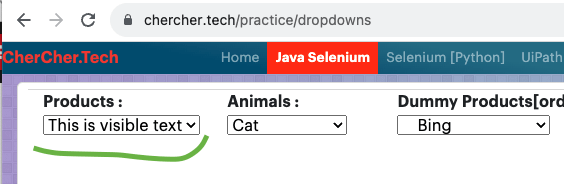
Select By Text with Playwright
page.locator("//select[@id='first']").select_option(label="Iphone")

Select By Index with Playwright
You can select the dropdown with index as well, the first option is index 0; I would strongly recommend against using this, because if the developer introduces another option in-between then the test fails.
page.locator("//select[@id='first']").select_option(index=1)

Get the currently selected Value:
We can get the currently selected value in playwright, based on the received value you can perform further operations.
Note: fetches the value of the option, not the text
from playwright.sync_api import sync_playwright
playwright = sync_playwright().start()
browser = playwright.chromium.launch(headless=False)
page = browser.new_page()
page.goto("https://chercher.tech/practice/dropdowns")
dropdown_options_selected = page.locator("select#first").input_value()
print(dropdown_options_selected)
#output
Microsoft
Get all options present in the dropdown
First point your locator to the dropdown options, if you have more than one dropdown make sure you form the locator that covers the dropdown as well. For example, your dropdown has an ID as first
and then options are with option
tag. then form CSS locator #first option
or XPATH //*[@id='first']/option
from playwright.sync_api import sync_playwright
playwright = sync_playwright().start()
browser = playwright.chromium.launch(headless=False)
page = browser.new_page()
page.goto("https://chercher.tech/practice/dropdowns")
dropdown_options = page.locator("#first option").all_text_contents()
print(dropdown_options)

Check whether an option present in the dropdown:
You can use the same above code just add an assertion.
dropdown_options = page.locator("#first option").all_text_contents()
print(dropdown_options)
assert "Iphone" in dropdown_options
Select By element with the Playwright
Find the option element using page.locator("").element_handle()
then pass it to select_option
function.
from playwright.sync_api import sync_playwright
playwright = sync_playwright().start()
browser = playwright.chromium.launch(headless=False)
page = browser.new_page()
page.goto("https://chercher.tech/practice/dropdowns")
option_to_select = page.locator("(//option[@value='Apple'])[1]").element_handle()
page.locator("//select[@id='first']").select_option(element=option_to_select)
Select dropdown value using Plawright Javascript
You can also use JavaScript to select a value from a dropdown, this will work only with the standard dropdowns.
But How do you know what query to use, Actually you do not. You have to do trial and error on the console tab in dev tools. We have a chapter for this, please learn about queries to execute on the console.
from playwright.sync_api import sync_playwright
playwright = sync_playwright().start()
browser = playwright.chromium.launch(headless=False)
page = browser.new_page()
page.goto("https://chercher.tech/practice/dropdowns")
page.evaluate("document.querySelector('[value=Yah]').selected=true")

Handle custom dropdown with Click:
Custom dropdowns are formed with application-building tools or drag-and-drop tools, most of the time they may not follow the HTML select tag. You can select a custom dropdown value using a few clicks.
- click the dropdown
- click the option from the list
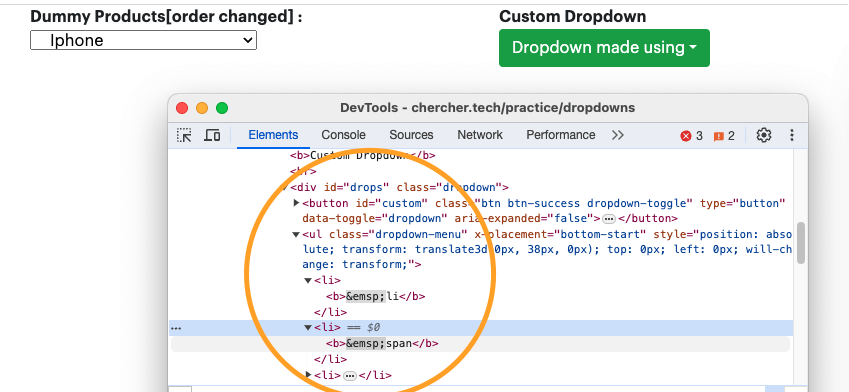
If you notice in the above one, it looks like a button but it is a dropdown, if you click the dropdown then you will see the options.
playwright = sync_playwright().start()
browser = playwright.chromium.launch(headless=False)
page = browser.new_page()
page.goto("https://chercher.tech/practice/dropdowns")
page.locator("#custom").click()
page.locator("//li/b[contains(text(), 'submenu')]")
Using Loop
Similar to the above one, we can use for loop to click the same, we will loop till we find text that we are interested in then we click it. Then close the loop.
from playwright.sync_api import sync_playwright
playwright = sync_playwright().start()
browser = playwright.chromium.launch(headless=False)
page = browser.new_page()
page.goto("https://chercher.tech/practice/dropdowns")
page.locator("#custom").click()
all_options = page.locator("#custom").all()
for option in all_options:
if "submenu" in option.text_content():
option.click()
break
Handle Multi Value dropdown with Playwright.
While selecting a single value we are providing a string for select_option
function, for selecting the multi-option dropdown, you should provide a list of options as a parameter to select multiple values.
To form multiple value dropdowns, developers use an attribute called multiple=true
in the select tag.
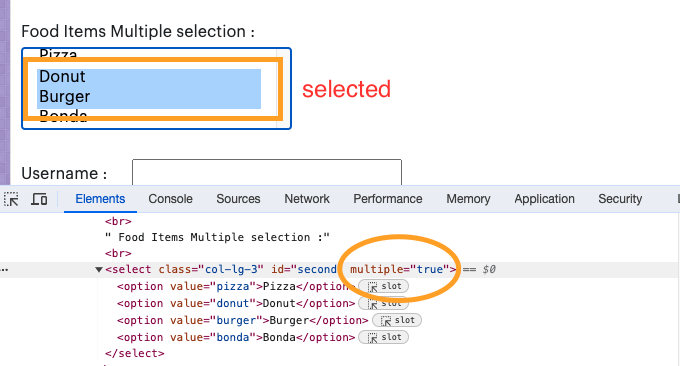
from playwright.sync_api import sync_playwright
playwright = sync_playwright().start()
browser = playwright.chromium.launch(headless=False)
page = browser.new_page()
page.goto("https://chercher.tech/practice/dropdowns")
page.locator("//select[@multiple='true']").select_option(label=["Donut", "Burger"])
Check whether a dropdown is a multiple dropdown:
is_multiple = page.locator("select#second").get_attribute("multiple")
print(is_multiple)
# output
true